Introduction
Like for any other Unity project, you’ll need a Camera in your level to see the action. The Corgi Engine includes a few Camera specific scripts. Note that you can use any Camera script with the asset, or implement your own, or build on top of the provided scripts. There’s nothing mandatory here and you can do whatever you want. This page covers the main scripts and how to use them.
Regular and UI Cameras
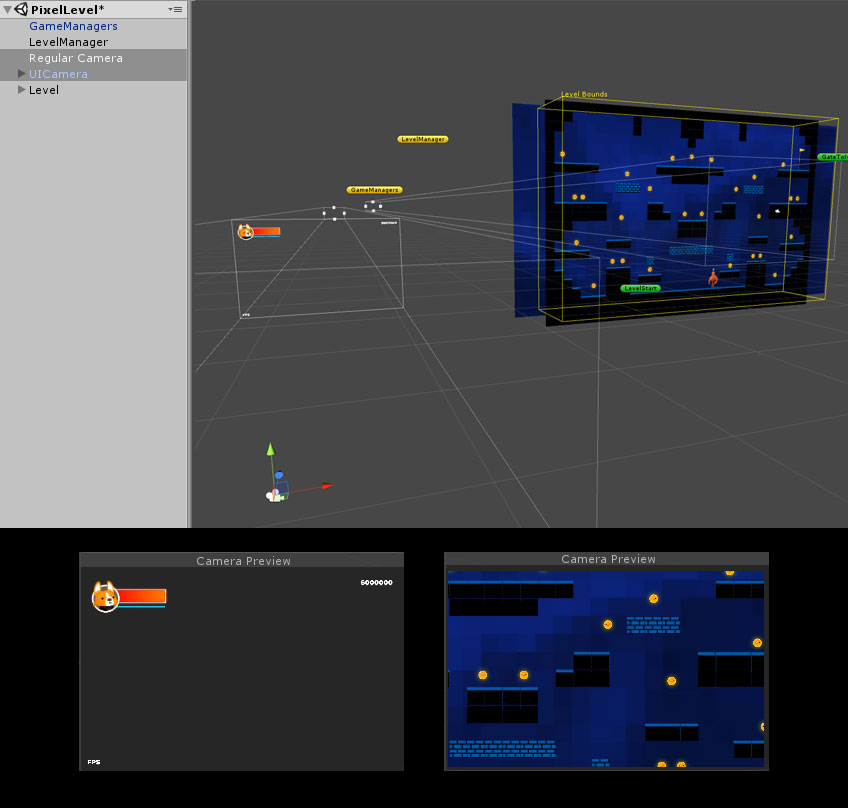
By default, in most demo scenes of the Corgi Engine, you’ll notice two cameras : a regular camera (2D, 3D, following the player or not, etc) and a UI Camera. The UI Camera’s Culling Mask is set on UI, which means it’ll only render UI tagged stuff, and is setup to be superimposed over the main camera’s render.
CameraController
The CameraController component can be added to any camera (orthographic or perspective), and it’ll make it move and try to follow the player. By default it’ll center on your main character (the first playable Character you’ll set in the scene’s LevelManager). From its inspector, you can define a few offsets, that will be added to this position depending on certain situations. The Camera offset will be applied at all times. The Horizontal Look Distance will be applied when moving left or right. Same thing for the up/down look distance. And you can also define with the LookAheadTrigger value how much you need to move for that offset to apply.
Then you can define movement speed and zoom values, with pretty self-explanatory settings. The final thing you can check is a checkbox that will automatically enable or disable effects on mobile. If you set it off, all standard assets effects added to the camera will be disabled when running on Android or iOS.
The asset also includes a multiplayer camera controller. It’s basically the same idea as the single player one, but it’ll track all playable characters in the scene.
Cinemachine
The Corgi Engine supports Cinemachine natively since v6.0. You’ll find examples of it in use in the Retro demo scenes (and others). This documentation won’t cover all the details of this amazing tool, head over to Cinemachine’s own documentation to learn more about it.
To add Cinemachine to your scene, you can simply go to the Cinemachine menu and create a new virtual camera. To make it work with the Corgi Engine, you’ll need to add a CinemachineCameraController component to it. In it you’ll be able to define whether you want it to follow the player, the expected speed of the player, orthographic or perspective zoom settings, etc. It’s relatively similar to the regular camera controller.
Pixel Perfect
From the CameraController’s inspector, you’ll also be able to set whether or not you want a pixel perfect camera. Most suited for 2D pixel art games, the pixel perfect camera will disable zoom, and instead determine a new orthographic camera size based on your settings in the inspector (the target vertical resolution at which for which you produced your sprites) and your desired PPU (pixels per unit) value.
Without this PixelPerfect setting, it can happen that a sprite is not rendered correctly, as each pixel of your sprite might not end up on one rounded screen pixel. To avoid this, here are a few things you can do :
- Create your assets for a target vertical resolution (768px for example), and set that value as your Reference Vertical Resolution in the CameraController’s inspector.
- For each of your sprites, define a common PPU value (32 for example), and set that value as your Reference Pixels Per Unit value in the CameraController’s inspector.
- On your sprites, set compression to None, and Filter Mode to Point (no filter).
You can also learn more about Pixel Perfect cameras in Unity in this blog post.
Parallax
The Corgi Engine includes everything you need to add parallax effects to your game. There are basically two ways to do it.
-
3D Parallax : the easiest way, built-in in Unity, is to have a 2.5D scene. Meaning you have a 3D scene and lateral movement from left to right. The Demos/Corgi3D/3DLevel demo scene is a good showcase of that. You don’t need anything in particular for it to work, just position some elements further from the camera than your main plane (the plane where the character moves) and they’ll move slower from left to right when your character moves (and the camera follows). Position them between the camera and your main plane, and they’ll appear to move faster when the camera moves.
-
2D Parallax : to achieve the same effect in 2D though, you’ll need the help of a few of the Corgi Engine’s scripts. First of all, you’ll need a Camera, with a CameraController script on top of it. You can assemble one yourself, or copy one from the Minimal demo scene. On that camera you need to add a ParallaxCamera component, and leave its MoveParallax checkbox checked. Then, on any gameobject in your scene that you want to move on sync with the camera movement, you can just add a ParallaxElement component.
There are a few options to setup there : vertical and horizontal speed (the higher the value, the faster it’ll move), and whether or not this should move in the opposite direction as the camera. In most cases you’ll want to leave that checked. Then you can either press play and check the result, or simply, while in editor mode, move the camera around and see how these elements react. This is useful for artists and level designers, you can check where a background element will be when the camera reaches a certain position. All that’s left to do is adjust speed values to give an impression of depth if you have more than one plane. For example, if you have 3 background elements : a tree, a mountain and the moon, respective values of 0.5, 0.3 and 0.1 should give a good impression of depth.
One last thing to consider is the LevelBackground script. Useful for 2D levels, it allows you to glue a sprite to the camera, and it’ll just follow it everywhere. Mostly used for skies.
Screen shakes
Screen shakes are the best. The engine is built with game feel at its core, and the MMFeedbacks library, included as a gift in the engine, is entirely dedicated to that.
There are multiple ways you can add screen shakes to your game in the engine, the recommended way is to use Cinemachine for your camera, and the Cinemachine Impulse system to handle screen shakes. This ties in nicely with the engine that supports it, and with MMFeedbacks which let you trigger them painlessly. You can learn how to setup Cinemachine cameras above on this very page, and you can learn how to use MMFeedbacks to trigger shakes in MMFeedbacks’ documentation.
Alternatively, you can use the legacy CameraController included in the engine, it also handles screen shakes, that can also be triggered via MMFeedbacks, or via events, like so :
MMCameraShakeEvent.Trigger(duration, amplitude, frequency, false, 0);
For example, this would trigger a relatively mild and short shake :
MMCameraShakeEvent.Trigger(0.05f, 1f, 0.02f, false, 0);
You can achieve the same result by creating a MMFeedbacks object in your scene, adding a CameraShake feedback to it, and having the same settings.
Lastly, you can use the MMCameraShaker component, which will work with the Wiggle component.
It’s recommended going the Cinemachine way, it’s by far the most Unity native, powerful and future proof of all these options. You’ll find examples of screen shakes in action in pretty much all the demos (the RetroCorgi ones for example). And you can learn more about MMFeedbacks on the dedicated website and the associated documentation. If you already own the Corgi Engine, do not buy MMFeedbacks twice, it’s already included in the engine as a gift already, no need to purchase it twice :)