Introduction
Once you’ve created your character, you’ll probably want to play with it. There are multiple ways to do it, and this page explains the different ways you can add characters, whether they’re playable or not, to your scene.
The Level Manager
The LevelManager class is a very central class in the Corgi Engine. It’s responsible for spawning the player(s), handling checkpoints as well as points of entry in a scene. It basically defines the rules of your level as a whole. It will also be used as the main way for classes throughout the engine to communicate with the player.
It’s recommended to have the LevelManager in charge of spawning your character. This means it won’t be present in the scene until your press Play, and will be instantiated at runtime. To let your LevelManager know what character it should instantiate, select the LevelManager object in your scene’s hierarchy, unfold the PlayerPrefabs array, and just drag and drop a Character prefab from your project view into the Element0 field.
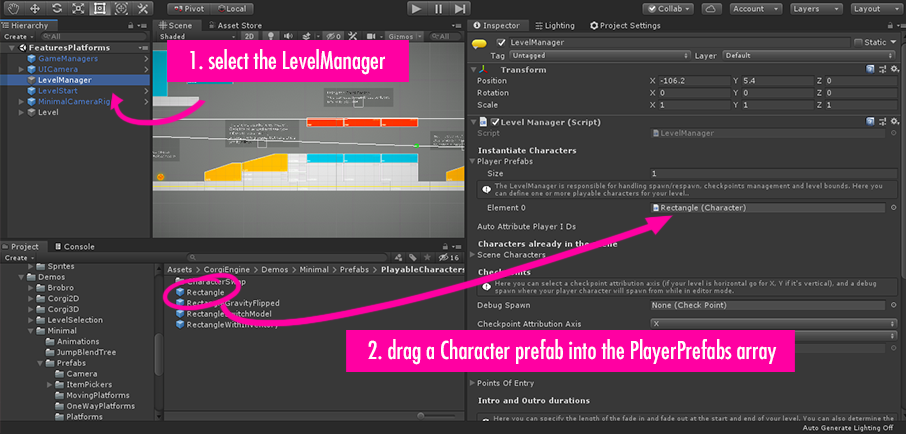
You can learn more about the LevelManager in the dedicated part of the documentation.
Having your character already present in your scene
While the method above is the recommended one, you can also have your character already present in your scene and still have the LevelManager reference it. For this to work, you’ll need to have a Character object in your scene, and drag it into the LevelManager’s SceneCharacters array.
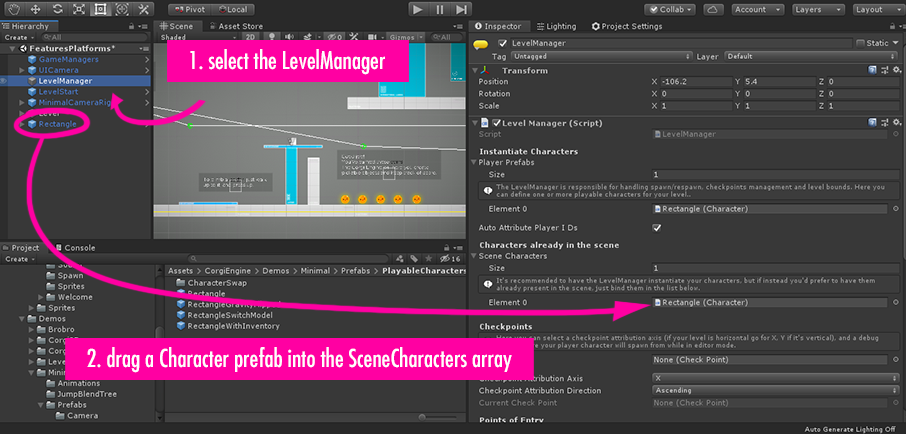
Other characters (AIs, NPCs)
For other characters, that are not meant to be controlled by the player, it’s much easier, you just have to drag their prefab somewhere in the scene.
Multiplayer
In multiplayer, you’ll have to let your MultiplayerLevelManager know what characters to instantiate. This is done just like for a single character :
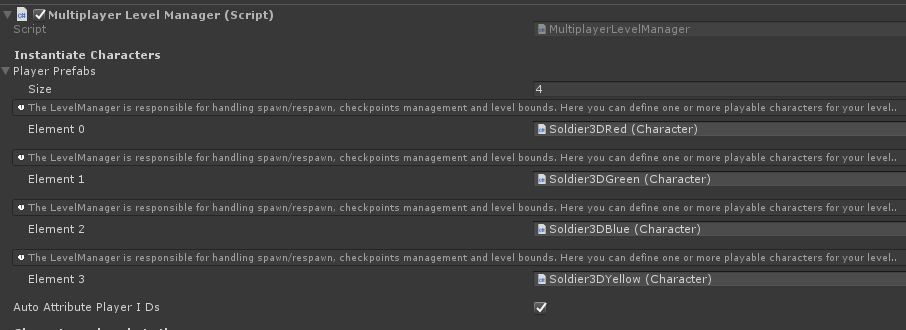
Accessing the player character via script
The best way to access the player(s) via script is via the LevelManager class, as it keeps a reference to it at all times (or to them if you have more than one playable character in your level). From any class, you can access the player array via :
LevelManager.Instance.Players
Players is an array, so if you only have one playable character, you can access it via :
LevelManager.Instance.Players[0]
And it’s an array of Characters, so from there it’s very easy to interact with your player :
// this will freeze your main character
LevelManager.Instance.Players[0].Freeze();
// sets the main character's max Health to 50
LevelManager.Instance.Players[0].gameObject.MMGetComponentNoAlloc<Health>().MaximumHealth = 50;
// forces the character to dash
LevelManager.Instance.Players[0].gameObject.MMGetComponentNoAlloc<CharacterDash>().StartDash();